How to Check if a Browser Extension is Installed Using AutoIt
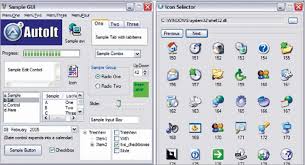
When working with web automation, one of the most common tasks is verifying whether a browser extension is installed. This is especially useful for automating workflows, testing, or ensuring that necessary extensions are available for your tasks. AutoIt, a scripting language primarily used for automating Windows GUI and general scripting tasks, can be leveraged to check if a browser extension is installed. However, checking for an extension’s presence depends on the browser you’re targeting.
In this article, we will dive into how to check if a browser extension is installed using AutoIt. We’ll discuss methods for popular browsers like Google Chrome and Mozilla Firefox, including how AutoIt can interact with their specific file structures and settings.
What is AutoIt?
AutoIt is a free scripting language designed for automating the Windows GUI and general scripting. It provides a simple way to simulate keystrokes, mouse movements, and window commands, making it ideal for automating repetitive tasks. AutoIt allows you to write small scripts that can handle tasks such as checking for the existence of files, interacting with applications, or controlling browser processes.
For our purposes, AutoIt can be used to automate the process of checking whether a browser extension is installed, specifically focusing on browser configurations and directories where extensions are stored.
Understanding Browser Extensions
Browser extensions are small software programs that customize the browsing experience by adding new features to a web browser. They can be installed from the browser’s extension store (such as Chrome Web Store or Firefox Add-ons) and are typically stored in the browser’s profile or extension directory.
Each browser handles extensions differently, so it’s important to understand how to check for their presence based on the browser being used.
READ ALSO: How to Check Your Mobile Network Speed
How to Check if a Browser Extension is Installed Using AutoIt in Google Chrome
Google Chrome stores installed extensions in a specific directory within the user profile. This is where we can check for the existence of an extension. Here’s how you can approach this task using AutoIt.
1. Locate Chrome’s Extension Directory
For Chrome, extensions are stored in the following directory:
- Windows 10/11:
C:\Users\<YourUsername>\AppData\Local\Google\Chrome\User Data\Default\Extensions
Each installed extension has a unique ID, which you can use to check if it’s installed. The ID is a long alphanumeric string assigned to the extension when it’s installed.
2. Using AutoIt to Check the Extension Directory
Here’s a simple AutoIt script to check if a specific Chrome extension is installed:
#include <File.au3> ; Define the Chrome extensions directory $chromeExtDir = "C:\Users\YourUsername\AppData\Local\Google\Chrome\User Data\Default\Extensions" ; Define the unique extension ID (replace with the actual ID of the extension you're checking for) $extensionID = "abcdefghijklmnopqrstuvwx" ; Build the path to the extension's directory $extensionPath = $chromeExtDir & "\" & $extensionID ; Check if the directory exists If FileExists($extensionPath) Then MsgBox(0, "Extension Status", "The extension is installed!") Else MsgBox(0, "Extension Status", "The extension is not installed.") EndIf
Explanation:
- FileExists: This function checks if the specified directory (the path to the extension) exists.
- $extensionID: Replace this with the unique ID of the extension you want to check.
- The script will display a message box telling you whether the extension is installed or not.
3. Getting the Extension ID
To get the extension ID in Chrome:
- Go to
chrome://extensions/
in the browser. - Enable Developer mode.
- Find your extension and copy the ID listed next to it.
SEE ALSO: Should You Accept Website Cache and Cookies?
How to Check for Extensions in Mozilla Firefox using AutoIt
Mozilla Firefox stores extensions in a slightly different way, using the profile directory. Firefox extensions are typically stored in the extensions
subdirectory of your Firefox profile.
1. Locate Firefox’s Extension Directory
For Firefox, extensions are stored in:
- Windows 10/11:
C:\Users\<YourUsername>\AppData\Roaming\Mozilla\Firefox\Profiles\<profile>\extensions
Here, <profile>
refers to your Firefox profile folder, and inside it, you will find subfolders for each extension.
2. Using AutoIt to Check the Extension Directory
Here’s a simple AutoIt script to check if a Firefox extension is installed:
#include <File.au3> ; Define the Firefox profile directory $firefoxProfileDir = "C:\Users\YourUsername\AppData\Roaming\Mozilla\Firefox\Profiles\YourProfile\extensions" ; Define the extension's ID (replace with the actual extension ID) $extensionID = "[email protected]" ; Build the path to the extension's directory $extensionPath = $firefoxProfileDir & "\" & $extensionID ; Check if the directory exists If FileExists($extensionPath) Then MsgBox(0, "Extension Status", "The extension is installed!") Else MsgBox(0, "Extension Status", "The extension is not installed.") EndIf
Explanation:
- Similar to Chrome, the script checks the presence of the extension’s ID in the
extensions
folder. - The message box will indicate if the extension is installed or not.
3. Getting the Extension ID
To find the ID of a Firefox extension:
- Open the
about:debugging
page in Firefox. - Click on This Firefox and find your extension.
- Click Inspect and you will see the extension’s ID listed in the URL.
READ ALSO: What’s New? See top 7 Latest Laptop Features
Handling Browser Extensions in Microsoft Edge
Microsoft Edge (Chromium-based) uses a similar structure to Google Chrome for extensions. Therefore, you can use the same approach as in Chrome to check for extensions in Edge. Edge’s extension directory is located here:
- Windows 10/11:
C:\Users\<YourUsername>\AppData\Local\Microsoft\Edge\User Data\Default\Extensions
You can follow the same script template as for Chrome, replacing chrome
with edge
and using the appropriate extension ID.
Potential Challenges
- Permissions: Ensure that your AutoIt script runs with sufficient permissions to access the directories where extensions are stored, particularly in Windows 10/11, where permissions may be restricted.
- Profile Variability: Users may have multiple browser profiles, and each profile could have a different set of extensions installed. Make sure you are targeting the correct profile.
- Dynamic Extensions: Some browsers, particularly Chrome and Edge, can update extensions dynamically or install them as part of a package, making it difficult to track them using file paths alone. You may need to use browser-specific APIs or developer tools to check extensions programmatically.
Conclusion
Now that you know how to use AutoIt to check for browser extensions, you can start automating your workflow. Whether you’re automating testing, setting up a deployment system, or just experimenting with AutoIt, these techniques will save you a lot of time. Don’t forget to test and refine your scripts to make sure they work across all browsers and extension types.
If you’ve tried using AutoIt for browser extension checks, let us know how it went! What challenges did you face? Feel free to comment below.